How to debug SvelteKit server-side code with Chromium DevTools
I have read this before:
Background
The SvelteKit Server is a node application. It is a node process.
And node is capable of exposing a debugging server, which we can connect right from our chromium based browsers like Chrome or Brave.
The way we do this is by passing the --inspect
or --inspect-brk
flag to the node
cli:
node --inspect index.js
Vite binary
During development, we run vite dev
to start the SvelteKit application, including the server and client parts.
If we now want to inspect the backend part of our SvelteKit server, we have to pass the inspect flag to the node process of our dev server.
The way binaries are managed by node package managers is the node_modules/.bin/…
directory.
We find a vite
executable.
Which is a copy of the one you find on GitHub.
Node Env
If your executable starts with:
#!/usr/bin/env node
The file actually contains JavaScript contents, and you can directly run it with node.
node --inspect node_modules/.bin/vite dev
You are probably using npm or yarn.
PNPM
If your executable starts with:
#!/bin/sh
The file is a shell script and when we execute it with node it will fail.
You are probably using PNPM, which generates shell scripts for binaries to link them correctly, the way PNPM structures dependencies.
Node options
Luckily, there is another way to pass the inspect flag to the node process we are targeting.
We can specify the environment variable NODE_OPTIONS
:
NODE_OPTIONS='--inspect' node_modules/.bin/vite dev
Attach debugger
Once we have run the command with the inspect option, we will get something like:
Debugger listening on ws://127.0.0.1:9229/0161c10f-86f3-4c06-9c20-351fb575df14
For help, see: https://nodejs.org/en/docs/inspector
This tells us that node is now running with a debugger.
We can connect to the debugger with a debugging client.
With ad chromium based browser, we already have a debugger built-in.
Chromium Debugger
Based on your browser, go to <browser>://inspect
.
Under the Remote Target you will find a list of running processes which expose a debugger.
We can connect by clicking Inspect near the path of the process.
This opens a fresh DevTools Window connected to our node process.
The stdout of our node process is directly connected to the console of the DevTools, so we can see all terminal messages right inside the DevTools console as well.
Debugging
To actually inspect and debug our server-side code, we can place the debugger
statement anywhere in our server-side code.
Normally, we can only use this statement within our client-side code and check out the regular DevTools of the webpage we are developing.
But since we are connected to our node process of our backend, we can also make use of it in our server-side code now.
Example
For example, when we have a +page.server.ts we can debug with:
// …
export const actions: Actions = {
default: async (event) => {
debugger
},
}
// …
This pauses executing on the line of the debugger and lets us inspect the state of code execution at this given point of time and a lot more useful possibilities.
In the above example, we can just inspect the event
variable of the local scope and see all properties.
Also, it's way better than just console.log()
because we have access to the complete local scope of the statement we paused execution.
There is no need to change the console.log()
content and jump back to the terminal again anymore.
We are everything nicely presented and directly inspectable in DevTools.
Wrap up
Add a debugging script to your package.json:
"dev:inspect": "NODE_OPTIONS='--inspect' vite dev",
Run the command:
pnpm run dev:inspect
Attach your browser as debugging client: Go to <browser>://inspect
and hit Inspect on the process started in the previous step.
Place the debugger
statement in your server-side code and start debugging right from DevTools.
Reference
- https://youtu.be/ubeOpB9FlZE
How do you debug SvelteKit server-side code?
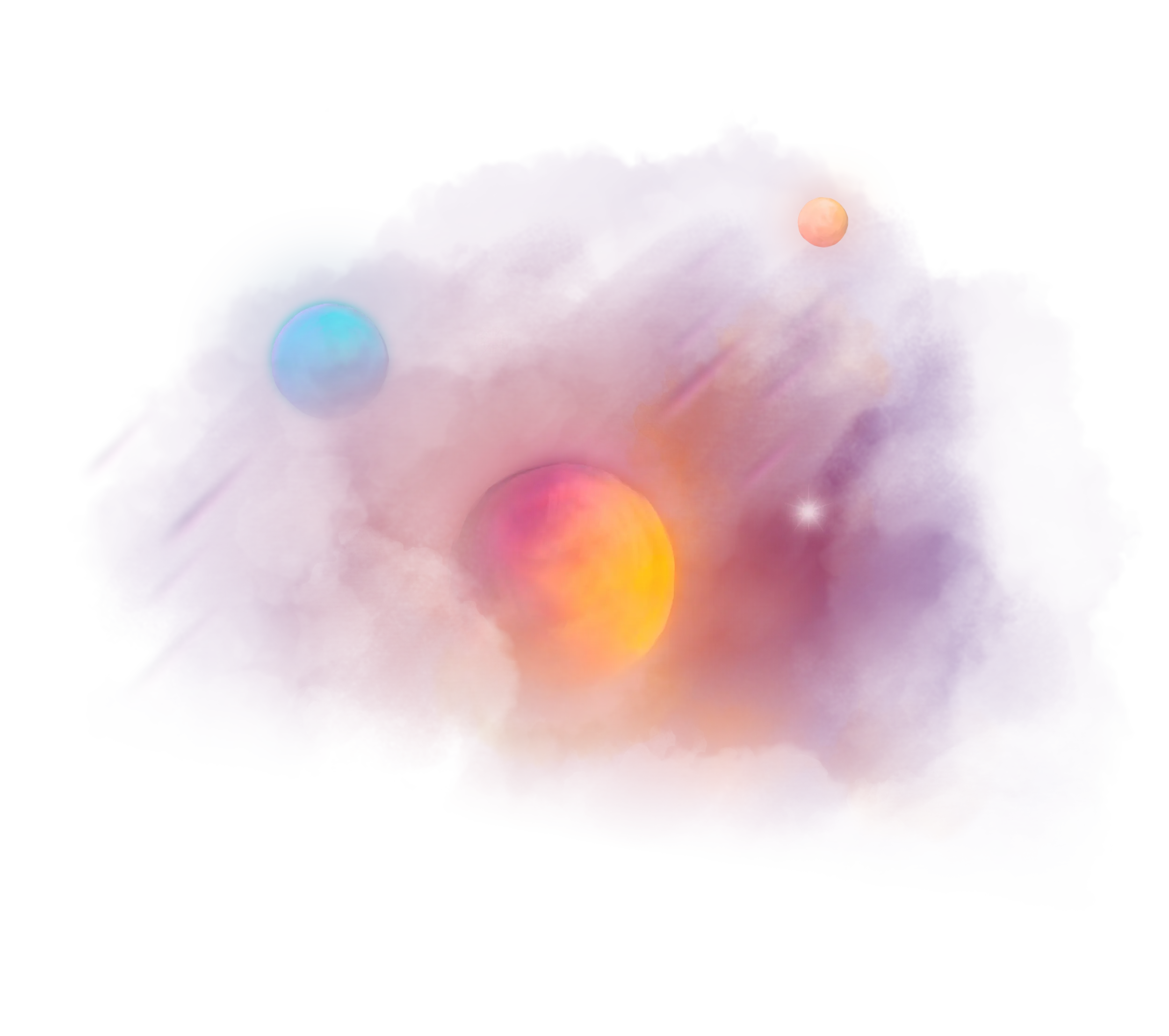